mirror of
https://github.com/prometheus/node_exporter.git
synced 2024-12-03 15:31:05 +01:00
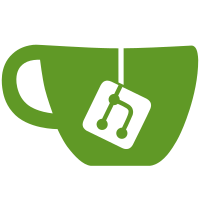
* add a map of profilers to CPUids `runtime.NumCPU()` returns the number of CPUs that the process can run on. This number does not necessarily correlate to CPU ids if the affinity mask of the process is set. This change maintains the current behavior as default, but also allows the user to specify a range of CPUids to use instead. The CPU id is stored as the value of a map keyed on the profiler object's address. Signed-off-by: Joe Damato <jdamato@fastly.com> Signed-off-by: Daniel Hodges <hodges.daniel.scott@gmail.com> Signed-off-by: Daniel Hodges <hodges@uber.com> Co-authored-by: jdamato-fsly <55214354+jdamato-fsly@users.noreply.github.com>
130 lines
2.8 KiB
Go
130 lines
2.8 KiB
Go
// Copyright 2019 The Prometheus Authors
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
// +build !noprocesses
|
|
|
|
package collector
|
|
|
|
import (
|
|
"github.com/go-kit/kit/log"
|
|
"io/ioutil"
|
|
"strconv"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/prometheus/client_golang/prometheus"
|
|
)
|
|
|
|
func TestPerfCollector(t *testing.T) {
|
|
paranoidBytes, err := ioutil.ReadFile("/proc/sys/kernel/perf_event_paranoid")
|
|
if err != nil {
|
|
t.Skip("Procfs not mounted, skipping perf tests")
|
|
}
|
|
paranoidStr := strings.Replace(string(paranoidBytes), "\n", "", -1)
|
|
paranoid, err := strconv.Atoi(paranoidStr)
|
|
if err != nil {
|
|
t.Fatalf("Expected perf_event_paranoid to be an int, got: %s", paranoidStr)
|
|
}
|
|
if paranoid >= 1 {
|
|
t.Skip("Skipping perf tests, set perf_event_paranoid to 0")
|
|
}
|
|
collector, err := NewPerfCollector(log.NewNopLogger())
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
// Setup background goroutine to capture metrics.
|
|
metrics := make(chan prometheus.Metric)
|
|
defer close(metrics)
|
|
go func() {
|
|
for range metrics {
|
|
}
|
|
}()
|
|
if err := collector.Update(metrics); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|
|
|
|
func TestPerfCPUFlagToCPUs(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
flag string
|
|
exCpus []int
|
|
errStr string
|
|
}{
|
|
{
|
|
name: "valid single cpu",
|
|
flag: "1",
|
|
exCpus: []int{1},
|
|
},
|
|
{
|
|
name: "valid range cpus",
|
|
flag: "1-5",
|
|
exCpus: []int{1, 2, 3, 4, 5},
|
|
},
|
|
{
|
|
name: "valid double digit",
|
|
flag: "10",
|
|
exCpus: []int{10},
|
|
},
|
|
{
|
|
name: "valid double digit range",
|
|
flag: "10-12",
|
|
exCpus: []int{10, 11, 12},
|
|
},
|
|
{
|
|
name: "valid double digit stride",
|
|
flag: "10-20:5",
|
|
exCpus: []int{10, 15, 20},
|
|
},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
t.Run(test.name, func(t *testing.T) {
|
|
cpus, err := perfCPUFlagToCPUs(test.flag)
|
|
if test.errStr != "" {
|
|
if err != nil {
|
|
t.Fatal("expected error to not be nil")
|
|
}
|
|
if test.errStr != err.Error() {
|
|
t.Fatalf(
|
|
"expected error %q, got %q",
|
|
test.errStr,
|
|
err.Error(),
|
|
)
|
|
}
|
|
return
|
|
}
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(cpus) != len(test.exCpus) {
|
|
t.Fatalf(
|
|
"expected cpus %v, got %v",
|
|
test.exCpus,
|
|
cpus,
|
|
)
|
|
}
|
|
for i := range cpus {
|
|
if test.exCpus[i] != cpus[i] {
|
|
t.Fatalf(
|
|
"expected cpus %v, got %v",
|
|
test.exCpus,
|
|
cpus,
|
|
)
|
|
}
|
|
}
|
|
})
|
|
}
|
|
}
|