mirror of
https://github.com/prometheus/node_exporter.git
synced 2024-12-20 07:26:08 +01:00
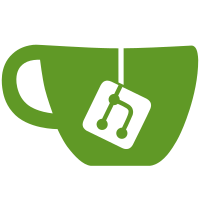
* Update vendor github.com/sirupsen/logrus@v1.1.1 * Update vendor github.com/coreos/go-systemd/dbus@v17 * Update vendor github.com/golang/protobuf/proto@v1.2.0 * Update vendor github.com/konsorten/go-windows-terminal-sequences@v1.0.1 * Update vendor github.com/mdlayher/... * Update vendor github.com/prometheus/procfs/... * Update vendor golang.org/x/... Signed-off-by: Ben Kochie <superq@gmail.com>
28 lines
759 B
Go
28 lines
759 B
Go
//+build linux
|
|
|
|
package netlink
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
"golang.org/x/sys/unix"
|
|
)
|
|
|
|
// getThreadNetNS gets the network namespace file descriptor of the thread the current goroutine
|
|
// is running on. Make sure to call runtime.LockOSThread() before this so the goroutine does not
|
|
// get scheduled on another thread in the meantime.
|
|
func getThreadNetNS() (int, error) {
|
|
file, err := os.Open(fmt.Sprintf("/proc/%d/task/%d/ns/net", unix.Getpid(), unix.Gettid()))
|
|
if err != nil {
|
|
return -1, err
|
|
}
|
|
return int(file.Fd()), nil
|
|
}
|
|
|
|
// setThreadNetNS sets the network namespace of the thread of the current goroutine to
|
|
// the namespace described by the user-provided file descriptor.
|
|
func setThreadNetNS(fd int) error {
|
|
return unix.Setns(fd, unix.CLONE_NEWNET)
|
|
}
|