mirror of
https://github.com/semaphoreui/semaphore.git
synced 2025-01-21 07:49:34 +01:00
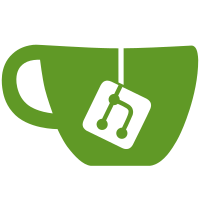
Changed the way the cloneInventoryRepo() function handles git repositories: The function will now try to pull the git repo if it can be pulled, else it will be cloned. This behaviour is based on what is done in the updateRepository() function Renamed the file from "LobalJob_inventory.go" to "LocalJob_inventory.go"
113 lines
2.5 KiB
Go
113 lines
2.5 KiB
Go
package tasks
|
|
|
|
import (
|
|
"os"
|
|
"path"
|
|
"strconv"
|
|
|
|
"github.com/ansible-semaphore/semaphore/db"
|
|
"github.com/ansible-semaphore/semaphore/db_lib"
|
|
log "github.com/sirupsen/logrus"
|
|
|
|
"github.com/ansible-semaphore/semaphore/util"
|
|
)
|
|
|
|
func (t *LocalJob) installInventory() (err error) {
|
|
if t.Inventory.SSHKeyID != nil {
|
|
t.sshKeyInstallation, err = t.Inventory.SSHKey.Install(db.AccessKeyRoleAnsibleUser, t.Logger)
|
|
if err != nil {
|
|
return
|
|
}
|
|
}
|
|
|
|
if t.Inventory.BecomeKeyID != nil {
|
|
t.becomeKeyInstallation, err = t.Inventory.BecomeKey.Install(db.AccessKeyRoleAnsibleBecomeUser, t.Logger)
|
|
if err != nil {
|
|
return
|
|
}
|
|
}
|
|
|
|
if t.Inventory.Type == db.InventoryFile {
|
|
err = t.cloneInventoryRepo()
|
|
} else if t.Inventory.Type == db.InventoryStatic || t.Inventory.Type == db.InventoryStaticYaml {
|
|
err = t.installStaticInventory()
|
|
}
|
|
|
|
return
|
|
}
|
|
|
|
func (t *LocalJob) tmpInventoryFilename() string {
|
|
return "inventory_" + strconv.Itoa(t.Task.ID)
|
|
}
|
|
|
|
func (t *LocalJob) tmpInventoryFullPath() string {
|
|
pathname := path.Join(util.Config.TmpPath, t.tmpInventoryFilename())
|
|
if t.Inventory.Type == db.InventoryStaticYaml {
|
|
pathname += ".yml"
|
|
}
|
|
return pathname
|
|
}
|
|
|
|
func (t *LocalJob) cloneInventoryRepo() error {
|
|
if t.Inventory.Repository == nil {
|
|
return nil
|
|
}
|
|
|
|
t.Log("cloning inventory repository")
|
|
|
|
repo := db_lib.GitRepository{
|
|
Logger: t.Logger,
|
|
TmpDirName: t.tmpInventoryFilename(),
|
|
Repository: *t.Inventory.Repository,
|
|
Client: db_lib.CreateDefaultGitClient(),
|
|
}
|
|
|
|
// Try to pull the repo before trying to clone it
|
|
if repo.CanBePulled() {
|
|
err := repo.Pull()
|
|
if err == nil {
|
|
return nil
|
|
}
|
|
}
|
|
|
|
err := os.RemoveAll(repo.GetFullPath())
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return repo.Clone()
|
|
}
|
|
|
|
func (t *LocalJob) installStaticInventory() error {
|
|
t.Log("installing static inventory")
|
|
|
|
path := t.tmpInventoryFullPath()
|
|
|
|
// create inventory file
|
|
return os.WriteFile(path, []byte(t.Inventory.Inventory), 0664)
|
|
}
|
|
|
|
func (t *LocalJob) destroyInventoryFile() {
|
|
path := t.tmpInventoryFullPath()
|
|
if err := os.Remove(path); err != nil {
|
|
log.Error(err)
|
|
}
|
|
}
|
|
|
|
func (t *LocalJob) destroyKeys() {
|
|
err := t.sshKeyInstallation.Destroy()
|
|
if err != nil {
|
|
t.Log("Can't destroy inventory user key, error: " + err.Error())
|
|
}
|
|
|
|
err = t.becomeKeyInstallation.Destroy()
|
|
if err != nil {
|
|
t.Log("Can't destroy inventory become user key, error: " + err.Error())
|
|
}
|
|
|
|
err = t.vaultFileInstallation.Destroy()
|
|
if err != nil {
|
|
t.Log("Can't destroy inventory vault password file, error: " + err.Error())
|
|
}
|
|
}
|