mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-11-23 20:37:12 +01:00
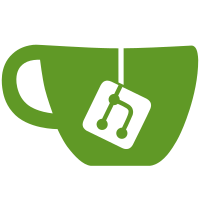
* app/vmctl: add verbose output for docker installations or when TTY isn't available * app/vmctl: fix tests * app/vmctl: make vmctl interactive if no tty * app/vmctl: cleanup * app/vmctl: add comment --------- Co-authored-by: Nikolay <nik@victoriametrics.com>
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
// Package barpool provides access to the global
|
|
// pool of progress bars, so they could be rendered
|
|
// altogether.
|
|
package barpool
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/app/vmctl/terminal"
|
|
"github.com/cheggaaa/pb/v3"
|
|
)
|
|
|
|
var pool = pb.NewPool()
|
|
|
|
// Add adds bar to the global pool
|
|
func Add(bar *pb.ProgressBar) { pool.Add(bar) }
|
|
|
|
// Start starts the global pool
|
|
// Must be called after all progress bars were added
|
|
func Start() error { return pool.Start() }
|
|
|
|
// Stop stops the global pool
|
|
func Stop() { _ = pool.Stop() }
|
|
|
|
// AddWithTemplate adds bar with the given template
|
|
// to the global pool
|
|
func AddWithTemplate(format string, total int) *pb.ProgressBar {
|
|
tpl := getTemplate(format)
|
|
bar := pb.ProgressBarTemplate(tpl).New(total)
|
|
Add(bar)
|
|
return bar
|
|
}
|
|
|
|
// NewSingleProgress returns progress bar with given template
|
|
func NewSingleProgress(format string, total int) *pb.ProgressBar {
|
|
tpl := getTemplate(format)
|
|
return pb.ProgressBarTemplate(tpl).New(total)
|
|
}
|
|
|
|
func getTemplate(format string) string {
|
|
isTerminal := terminal.IsTerminal(int(os.Stdout.Fd()))
|
|
if !isTerminal {
|
|
format = fmt.Sprintf("%s\n", format)
|
|
}
|
|
return format
|
|
}
|