mirror of
https://github.com/VictoriaMetrics/VictoriaMetrics.git
synced 2024-12-15 16:30:55 +01:00
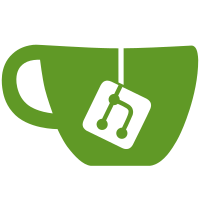
* started work on sd for k8s * continue work on watch sd * fixes * continue work * continue work on sd k8s * disable gzip * fixes typos * log errror * minor fix Co-authored-by: Aliaksandr Valialkin <valyala@gmail.com>
77 lines
1.6 KiB
Go
77 lines
1.6 KiB
Go
package kubernetes
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/VictoriaMetrics/VictoriaMetrics/lib/logger"
|
|
)
|
|
|
|
// SharedKubernetesCache holds cache of kubernetes objects for current config.
|
|
type SharedKubernetesCache struct {
|
|
Endpoints *sync.Map
|
|
EndpointsSlices *sync.Map
|
|
Pods *sync.Map
|
|
Services *sync.Map
|
|
}
|
|
|
|
// NewSharedKubernetesCache returns new cache.
|
|
func NewSharedKubernetesCache() *SharedKubernetesCache {
|
|
return &SharedKubernetesCache{
|
|
Endpoints: new(sync.Map),
|
|
EndpointsSlices: new(sync.Map),
|
|
Pods: new(sync.Map),
|
|
Services: new(sync.Map),
|
|
}
|
|
}
|
|
|
|
func updatePodCache(cache *sync.Map, p *Pod, action string) {
|
|
switch action {
|
|
case "ADDED":
|
|
cache.Store(p.key(), p)
|
|
case "DELETED":
|
|
cache.Delete(p.key())
|
|
case "MODIFIED":
|
|
cache.Store(p.key(), p)
|
|
case "ERROR":
|
|
default:
|
|
logger.Warnf("unexpected action: %s", action)
|
|
}
|
|
}
|
|
|
|
func updateServiceCache(cache *sync.Map, p *Service, action string) {
|
|
switch action {
|
|
case "ADDED", "MODIFIED":
|
|
cache.Store(p.key(), p)
|
|
case "DELETED":
|
|
cache.Delete(p.key())
|
|
case "ERROR":
|
|
default:
|
|
logger.Warnf("unexpected action: %s", action)
|
|
}
|
|
|
|
}
|
|
|
|
func updateEndpointsCache(cache *sync.Map, p *Endpoints, action string) {
|
|
switch action {
|
|
case "ADDED", "MODIFIED":
|
|
cache.Store(p.key(), p)
|
|
case "DELETED":
|
|
cache.Delete(p.key())
|
|
case "ERROR":
|
|
default:
|
|
logger.Warnf("unexpected action: %s", action)
|
|
}
|
|
}
|
|
|
|
func updateEndpointsSliceCache(cache *sync.Map, p *EndpointSlice, action string) {
|
|
switch action {
|
|
case "ADDED", "MODIFIED":
|
|
cache.Store(p.key(), p)
|
|
case "DELETED":
|
|
cache.Delete(p.key())
|
|
case "ERROR":
|
|
default:
|
|
logger.Infof("unexpected action: %s", action)
|
|
}
|
|
}
|