mirror of
https://github.com/xcp-ng/xenadmin.git
synced 2024-11-25 06:16:37 +01:00
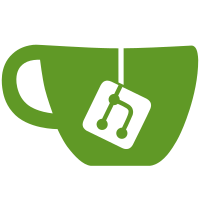
* CA-34935 ConnectionOptions invalid tooltip now dissappears when page changes or when the dialog moves Signed-off-by: Christopher Lancaster <christopher.lancaste1@citrix.com> * CA-349435 modified IOptionsPage & IEditPage to include a Hide Tooltip method, implemented it in all relevant classes. Hooked up events in the options dialog & properties dialog to hide tooltips on tab change or dialog move Signed-off-by: Christopher Lancaster <christopher.lancaste1@citrix.com> * CA-349435 removed duplicate pages that were added accidently/automatically Signed-off-by: Christopher Lancaster <christopher.lancaste1@citrix.com>
124 lines
3.6 KiB
C#
124 lines
3.6 KiB
C#
/* Copyright (c) Citrix Systems, Inc.
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms,
|
|
* with or without modification, are permitted provided
|
|
* that the following conditions are met:
|
|
*
|
|
* * Redistributions of source code must retain the above
|
|
* copyright notice, this list of conditions and the
|
|
* following disclaimer.
|
|
* * Redistributions in binary form must reproduce the above
|
|
* copyright notice, this list of conditions and the
|
|
* following disclaimer in the documentation and/or other
|
|
* materials provided with the distribution.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND
|
|
* CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
|
|
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
|
|
* MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
|
|
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
|
|
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
|
|
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
|
|
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
|
|
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
|
|
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
|
|
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
|
|
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
|
|
* SUCH DAMAGE.
|
|
*/
|
|
|
|
using System;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Windows.Forms;
|
|
using XenAdmin.Actions;
|
|
using XenAdmin.Core;
|
|
using XenAdmin.SettingsPanels;
|
|
using XenAPI;
|
|
|
|
|
|
namespace XenAdmin.Controls
|
|
{
|
|
public partial class UpsellPage : UserControl, IEditPage
|
|
{
|
|
public UpsellPage()
|
|
{
|
|
InitializeComponent();
|
|
this.LearnMoreButton.Visible = !HiddenFeatures.LearnMoreButtonHidden;
|
|
}
|
|
|
|
public void enableOkButton()
|
|
{
|
|
OKButton.Visible = true;
|
|
}
|
|
|
|
public string BlurbText
|
|
{
|
|
set => Blurb.Text = HiddenFeatures.LinkLabelHidden
|
|
? value
|
|
: value + string.Format(Messages.UPSELL_BLURB_TRIAL, BrandManager.ProductBrand);
|
|
}
|
|
|
|
public string LearnMoreUrl { private get; set; } = InvisibleMessages.UPSELL_LEARNMOREURL_TRIAL;
|
|
|
|
private void LearnMoreButton_Clicked(object sender, EventArgs e)
|
|
{
|
|
NavigateTo(LearnMoreUrl);
|
|
}
|
|
|
|
private void NavigateTo(string url)
|
|
{
|
|
if (url == null)
|
|
return;
|
|
Program.OpenURL(url);
|
|
}
|
|
|
|
#region IEditPage Members
|
|
|
|
public AsyncAction SaveSettings()
|
|
{
|
|
return null;
|
|
}
|
|
|
|
public void SetXenObjects(IXenObject orig, IXenObject clone)
|
|
{
|
|
}
|
|
|
|
public bool ValidToSave => true;
|
|
|
|
public void ShowLocalValidationMessages()
|
|
{
|
|
}
|
|
|
|
public void HideLocalValidationMessages()
|
|
{
|
|
}
|
|
|
|
public void Cleanup()
|
|
{
|
|
}
|
|
|
|
public bool HasChanged => false;
|
|
|
|
|
|
#region IVerticalTab Members
|
|
|
|
public string SubText => Messages.XENSERVER_UPGRADE_REQUIRED;
|
|
|
|
[Browsable(false)]
|
|
[DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)]
|
|
public Image Image { get; set; } = Images.StaticImages.Logo; //serving as default value; never really shown
|
|
|
|
#endregion
|
|
|
|
#endregion
|
|
|
|
private void OKButton_Click(object sender, EventArgs e)
|
|
{
|
|
ParentForm?.Close();
|
|
}
|
|
}
|
|
}
|