mirror of
https://github.com/xcp-ng/xenadmin.git
synced 2024-12-22 08:26:03 +01:00
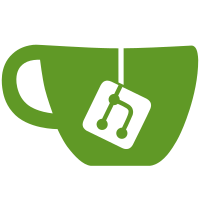
Implemented error handling. If there has been an error in a pool, we stop executing actions in that pool immediately, and report the error once every other actions have finished. Error in one pool will not affect the upgrade process of other pools. Signed-off-by: Gabor Apati-Nagy <gabor.apati-nagy@citrix.com>
54 lines
1.5 KiB
C#
54 lines
1.5 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Linq;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
using XenAdmin.Wizards.PatchingWizard.PlanActions;
|
|
using XenAPI;
|
|
|
|
namespace XenAdmin.Wizards.PatchingWizard
|
|
{
|
|
class UpdateProgressBackgroundWorker : BackgroundWorker
|
|
{
|
|
public List<PlanAction> PlanActions { get; private set; }
|
|
public List<PlanAction> DelayedActions { get; private set; }
|
|
private Host master;
|
|
|
|
public List<PlanAction> FinsihedActions = new List<PlanAction>();
|
|
public PlanAction FailedWithExceptionAction = null;
|
|
public List<PlanAction> doneActions = new List<PlanAction>();
|
|
|
|
public UpdateProgressBackgroundWorker(Host master, List<PlanAction> planActions, List<PlanAction> delayedActions)
|
|
{
|
|
this.master = master;
|
|
this.PlanActions = planActions;
|
|
this.DelayedActions = delayedActions;
|
|
}
|
|
|
|
public List<PlanAction> AllActions
|
|
{
|
|
get
|
|
{
|
|
return PlanActions.Concat(DelayedActions).ToList();
|
|
}
|
|
}
|
|
|
|
public int TotalNumberOfActions
|
|
{
|
|
get
|
|
{
|
|
return PlanActions.Count + DelayedActions.Count;
|
|
}
|
|
}
|
|
|
|
public int ProgressPercent
|
|
{
|
|
get
|
|
{
|
|
return (int)(1.0 / (double)TotalNumberOfActions * (double)(FinsihedActions.Count));
|
|
}
|
|
}
|
|
}
|
|
}
|