mirror of
https://github.com/xcp-ng/xenadmin.git
synced 2024-12-23 00:46:03 +01:00
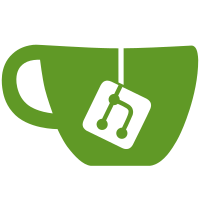
PVS Tab: - Move the "View PVS sites" button inside the "Configure PVS Cache" dialog (next to Remove button) and make it per-site (showing only the PVS servers from that site) - Remove "Currently attached" column from the VMs section - Rename "Status" column to "Read caching status" Configure PVS Cache" dialog: - Make sure there is a scrollbar to accommodate all hosts in a pool - Add a confirmation dialog when a site is removed. The action is executed when the Remove button is pressed. - When Memory SR is selected, set the maximum value to the dom0-extra-memory.. - Allow empty configuration (but see next point): The OK button should only be disabled if the PVS site name is empty - Add an extra option in the "Cache storage" combobox, with text "Not configured", selected by default on a new site. When this is selected, we will remove the existing entry in pvs_cache_storage and will not create a new one. - When a PVS site is deleted (forgotten), we first delete all the associated PVS_servers, otherwise the site cannot be deleted.
44 lines
1.6 KiB
C#
44 lines
1.6 KiB
C#
using System;
|
|
using System.Linq;
|
|
using XenAPI;
|
|
|
|
namespace XenAdmin.Actions
|
|
{
|
|
public class DeletePvsSiteAction : PureAsyncAction
|
|
{
|
|
private readonly PVS_site pvsSite;
|
|
|
|
public DeletePvsSiteAction(PVS_site pvsSite)
|
|
: base(pvsSite.Connection, string.Format(Messages.ACTION_DELETE_PVS_SITE_TITLE, pvsSite.Name.Ellipsise(50)),
|
|
Messages.ACTION_DELETE_PVS_SITE_DESCRIPTION, false)
|
|
{
|
|
System.Diagnostics.Trace.Assert(pvsSite != null);
|
|
this.pvsSite = pvsSite;
|
|
}
|
|
|
|
protected override void Run()
|
|
{
|
|
// check if there are any running proxies
|
|
var pvsProxies = Connection.Cache.PVS_proxies.Where(s => s.site.opaque_ref == pvsSite.opaque_ref).ToList();
|
|
if (pvsProxies.Count > 0)
|
|
{
|
|
throw new Failure(Failure.PVS_SITE_CONTAINS_RUNNING_PROXIES);
|
|
}
|
|
|
|
// delete PVS_servers
|
|
var pvsServers = Connection.Cache.PVS_servers.Where(s => s.site.opaque_ref == pvsSite.opaque_ref).ToList();
|
|
int inc = pvsServers.Count > 0 ? 50 / pvsServers.Count : 50;
|
|
foreach (var pvsServer in pvsServers)
|
|
{
|
|
RelatedTask = PVS_server.async_forget(Session, pvsServer.opaque_ref);
|
|
PollToCompletion(PercentComplete, PercentComplete + inc);
|
|
}
|
|
|
|
RelatedTask = PVS_site.async_forget(Session, pvsSite.opaque_ref);
|
|
PollToCompletion();
|
|
Description = Messages.ACTION_DELETE_PVS_SITE_DONE;
|
|
PercentComplete = 100;
|
|
}
|
|
}
|
|
}
|