mirror of
https://github.com/xcp-ng/xenadmin.git
synced 2024-11-24 22:06:59 +01:00
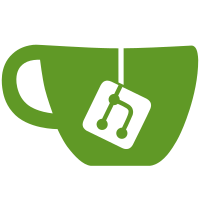
* CA-375900: Prepend `//?/` to file paths when creating streams for archive generation The string works to enable creation of files with paths larger than 260 characters. * CA-375900: Add directory support and rename utility method * Fix whitespace in `ArchiveWriterTest` * CA-375900: Explicitly enumerate files and directories in `ArchiveWriter` `Directory.GetFiles` and `Directory.GetDirectories` do not enumerate if paths are longer than 260, even when prepended with `//?/`. * CA-375900: Add long path tests to `ArchiveWriter` * CA-375900: Add long path tests to `ArchiveIterator` * CA-375900: Ensure files are added to folders in archive * Use a recursive method to add directories and files to archive in `ArchiveIterator` Also improves progress reporting by basing it on directory count * Fix typos * Expand `ArchiveWriterTests` to cover all combinations of directory and path lengths * Ensure that directories used in recursive `Directory.Delete` calls are using long path format If files in the directory exceed the 260 character limit, the calls will fail * Expand `ArchiveIteratorTests` to cover all combinations of directory and path lengths * Ensure relative path name removes `rootPath` * Fix typo * Do not use long paths when importing OVFs The import uses `DiscUtils` which cannot handle paths prepended with `//?/` * Remove use of `ToLongWindowsPath` within appliance export This partially reverts commit 819425855c56c14b937849714b359003465bd2f4. * Refactoring and some corrections. Signed-off-by: Danilo Del Busso <danilo.delbusso@cloud.com> Signed-off-by: Konstantina Chremmou <Konstantina.Chremmou@cloud.com> Co-authored-by: Konstantina Chremmou <Konstantina.Chremmou@cloud.com>
125 lines
4.2 KiB
C#
125 lines
4.2 KiB
C#
/* Copyright (c) Cloud Software Group, Inc.
|
|
*
|
|
* Redistribution and use in source and binary forms,
|
|
* with or without modification, are permitted provided
|
|
* that the following conditions are met:
|
|
*
|
|
* * Redistributions of source code must retain the above
|
|
* copyright notice, this list of conditions and the
|
|
* following disclaimer.
|
|
* * Redistributions in binary form must reproduce the above
|
|
* copyright notice, this list of conditions and the
|
|
* following disclaimer in the documentation and/or other
|
|
* materials provided with the distribution.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND
|
|
* CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
|
|
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
|
|
* MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
|
|
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
|
|
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
|
|
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
|
|
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
|
|
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
|
|
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
|
|
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
|
|
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
|
|
* SUCH DAMAGE.
|
|
*/
|
|
|
|
using System;
|
|
using System.IO;
|
|
using System.Reflection;
|
|
using System.Text;
|
|
using ICSharpCode.SharpZipLib.Tar;
|
|
|
|
namespace XenCenterLib.Archive
|
|
{
|
|
public class TarArchiveWriter : ArchiveWriter
|
|
{
|
|
private static readonly log4net.ILog log = log4net.LogManager.GetLogger(MethodBase.GetCurrentMethod().DeclaringType);
|
|
|
|
private TarOutputStream tar = null;
|
|
private const long bufferSize = 32 * 1024;
|
|
private bool disposed;
|
|
|
|
/// <summary>
|
|
/// Parameterless constructor needed by tests
|
|
/// </summary>
|
|
public TarArchiveWriter()
|
|
{
|
|
}
|
|
|
|
public TarArchiveWriter(Stream outputStream)
|
|
{
|
|
tar = new TarOutputStream(outputStream);
|
|
disposed = false;
|
|
}
|
|
|
|
public override void SetBaseStream(Stream outputStream)
|
|
{
|
|
tar = new TarOutputStream(outputStream);
|
|
disposed = false;
|
|
}
|
|
|
|
public override void AddDirectory(string directoryName, DateTime modificationTime)
|
|
{
|
|
StringBuilder sb = new StringBuilder(directoryName);
|
|
|
|
//Need to add a terminal front-slash to add a directory
|
|
if (!directoryName.EndsWith("/"))
|
|
sb.Append("/");
|
|
TarEntry entry = TarEntry.CreateTarEntry(sb.ToString());
|
|
entry.ModTime = modificationTime;
|
|
|
|
tar.PutNextEntry(entry);
|
|
tar.CloseEntry();
|
|
}
|
|
|
|
public override void Add(Stream fileToAdd, string fileName, DateTime modificationTime, Action cancellingDelegate)
|
|
{
|
|
TarEntry entry = TarEntry.CreateTarEntry(fileName);
|
|
entry.Size = fileToAdd.Length;
|
|
entry.ModTime = modificationTime;
|
|
|
|
tar.PutNextEntry(entry);
|
|
byte[] buffer = new byte[bufferSize];
|
|
int n;
|
|
|
|
//You have to do this because if using a memory stream the pointer will be at the end it
|
|
//it's just been read and this will cause nothing to be written out
|
|
fileToAdd.Position = 0;
|
|
|
|
while ((n = fileToAdd.Read(buffer, 0, buffer.Length)) > 0)
|
|
{
|
|
cancellingDelegate?.Invoke();
|
|
tar.Write(buffer, 0, n);
|
|
}
|
|
|
|
tar.Flush();
|
|
tar.CloseEntry();
|
|
}
|
|
|
|
protected override void Dispose(bool disposing)
|
|
{
|
|
if (!disposed && disposing)
|
|
{
|
|
try
|
|
{
|
|
tar?.Dispose();
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
//workaround for CA-347483
|
|
log.Error("Failed to dispose tar output stream", e);
|
|
}
|
|
|
|
disposed = true;
|
|
}
|
|
|
|
base.Dispose(disposing);
|
|
}
|
|
}
|
|
}
|